This section explains the internal representation and value area of each type for the data handled by the CC-RL.
The leftmost bit in an area is a sign bit with a signed type. The value of a signed type is expressed as 2' s complement.

Only the 0th bit has meaning. Bits 1 to 7 are undefined.
When the -lang=c and -strict_std options are specified, _Bool type will cause a C90 violation error.

A plain char type not specified as signed or unsigned has the same representation as unsigned char.
When the -signed_char option is used, a plain char type has the same representation as signed char.
signed char (no sign bit for unsigned)

short (no sign bit for unsigned)

int (no sign bit for unsigned)

long (no sign bit for unsigned)

long long (no sign bit for unsigned)

When the -lang=c and -strict_std options are specified, long long type will cause a C90 violation error.
The type of an integer constant will be the first type in the lists below capable of representing that value.
Types of Integer Constants (If type long long is enabled (when -lang=c is specified and -strict_std is not)) |
Different from C99 specification. This is added to avoid the case where an integer constant represented as 4-byte data in C90 is unexpectedly represented as 8-byte data. |
Types of Integer Constants (If type long long is disabled (when -lang=c and -strict_std are specified)) |
Internal Representation of floating-point data type conforms to IEC 60559:1989 (IEEE 754-1985)Note. The leftmost bit in an area of a sign bit. If the value of this sign bit is 0, the data is a positive value; if it is 1, the data is a negative value.
IEEE: Institute of Electrical and Electronics Engineers |


When the option -dbl_size=4 is used, it has the same representation as type float. Even if you write type double, it will be treated as if you had written type float. Similarly, if you write long double, it will be treated as if you had written type float.
When the option dbl_size=8 is used, it is represented in 64 bits.
The internal representation of a near pointer is a 16-bit unsigned type and that of a far pointer is a 32-bit unsigned type.
The most significant byte of a far pointer is undetermined.
The internal representation of a null pointer constant (NULL) has a value of 0. (Note that the byte corresponding to the undefined byte of the far pointer is not always 0.)
Therefore, for both the near and far pointers, access to address 0 is not guaranteed.
Correct operation is not guaranteed if the value of a far pointer exceeds 0xfffff.
Do not allocate a function or a variable to address 0x0f0000 or access the address.


The internal representation of an enumerated type depends on the range of the enumerator value.
The internal representation of an array type arranges the elements of an array in the form that satisfies the alignment condition (alignment) of the elements
The internal representation of the array shown above is as follows.

In a single structure, members are allocated from the head of the structure in the order of declaration. The internal representation of a structure type arranges the elements of a structure in a form that satisfies the alignment condition of the elements.
The alignment condition for the largest member of a structure is used as the alignment condition for the whole of the structure. This rule is also applied recursively when members are structures or unions.
The size of a structure is a multiple of the "alignment condition for the whole of the structure". Therefore, this size includes the unused area that is created to guarantee the alignment condition of the next data when the end of a structure does not match the alignment condition of that structure.
The internal representation of the structure shown above is as follows.


The internal representation of the structure shown above is as follows.


For details on the structure packing specification, see "-pack".
The alignment condition for the largest member of a union is used as the alignment condition for the whole of the union. This rule is also applied recursively when members are structures or unions.
The internal representation of the union shown in the above example is as follows.
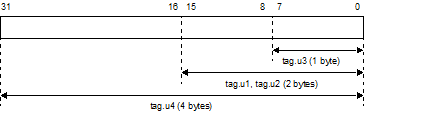
The types that can be specified for bit fields are _Bool, char, signed char, unsigned char, signed short, unsigned short, signed int, unsigned int, signed long, unsigned long, signed long long, unsigned long long, and enumerated types.
Although only (signed or unsigned) int is allowed in C90, all of the above types are valid for bit fields in CC-RL if the -strict_std option is not used.
With the -strict_std option, the _Bool, signed long long, and unsigned long long types will cause a C90 violation error.
A bit field is allocated starting from the least significant bit for the type specified in the declaration of the bit field.
When an attempt is made to allocate a bit field from the bit contiguous to the previous bit field and the location of the end of the bit field after allocation exceeds the location of adding the "bit width of declared type" to the boundary that is previous to satisfaction of the alignment condition for the bit field, the bit field is allocated to an area starting from the first boundary that satisfies the alignment condition after the previous bit field.
The bit field of a type not specified as signed or unsigned is handled as unsigned. |
Bit fields within an allocation unit are allocated from the lower order (LSB) toward the higher order (MSB). |
struct S{ char a; char b:2; signed char c:3; unsigned char d:4; int e; short f:5; int g:6; unsigned char h:2; unsigned int i:2; }; |
The internal representation for the bit field in the above example is as follows.
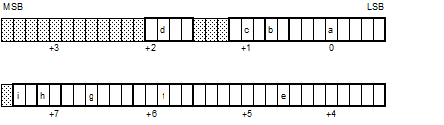
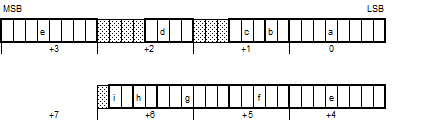
The internal representation for the bit field in the above example is as follows.

The internal representation for the bit field in the above example is as follows.


For details on the structure packing specification, see "-pack".
Alignment condition for basic type is as follows.
Alignment condition for enumerated type is as follows.
The alignment condition for an array type is the same as that for the array elements.
The alignment condition (value) for near and far pointers is 2.
The alignment conditions for a union type are the same as those of the structure's member whose type has the largest alignment condition.
The alignment conditions for a structure type are the same as those of the structure's member whose type has the largest alignment condition.
See "9.1 Function Call Interface".
The alignment condition for a function is a 1-byte boundary.